Shop Test
A downloadable game
A game created in ~5-6 hours for a one day game challenge as part of my Mastered Game Developer course.
The brief here was to create a shop system that you'd find inside of a game, with an extension of using some microtransaction plugins to experiment with some real word money spending.
You can find the Github Repo here: https://github.com/Woeger/Shop-Test
CONTROLS:
WASD: Movement
Mouse: Camera
CODE:
Below is some excepts from my Blueprints. The real interesting part about this project is how I stored and displayed the data. In essence thats what shops in video games boil down to, they're selling items which all have a price, description, effects... which is structured similarly to how you'd find a real life database of items. As a result I leveraged a lot of Unreal's data structures with some clever widget usage.
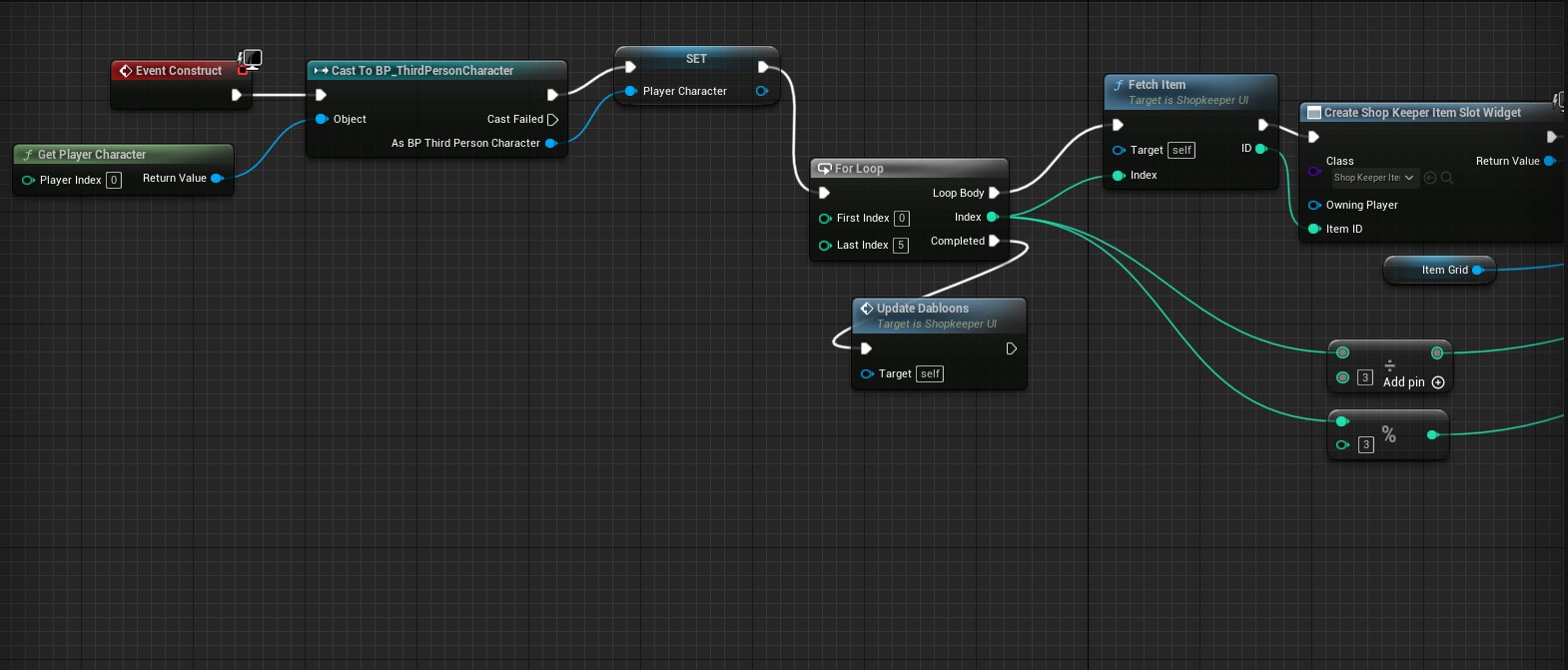
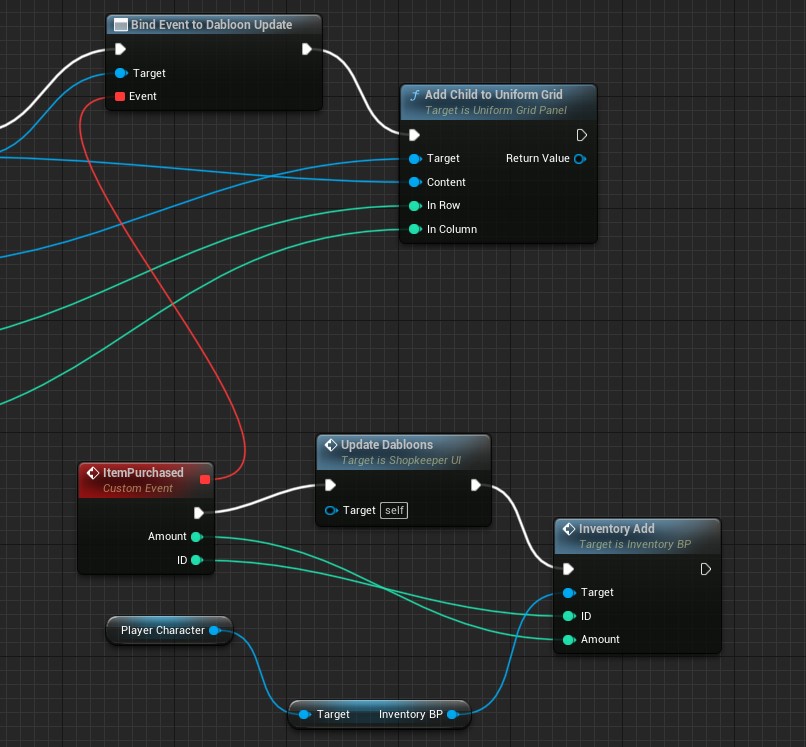
This snippet here is where most of the main logic happens. The first thing we do is get the player character and cast it to our BP_PlayerCharacter type and promote that to a variable. We need to do this as we edit our players inventory when buying items, so we need access to an instance of it.
Next is where the items are setup. A shopkeeper (who's logic is in a separate BP) has their own inventory which is stored as an array of items. We do a for loop to get the first six items they have. (In my game there exists only 6 items.) While this works for a prototype, it'd be better if it could dynamically adjust to the size of that array, in the future I'd get the length and plug that into our loop as the last index.
In that loop, we use a function I made called 'Fetch Item' which you can see below.
This is pretty simple. We get that array I talked about earlier, and take the index of our for loop, check that index is valid and if true we return the ID for that item. (Which is defined in our data structure). If this isn't valid, we return -1 which is not a valid ID and will be caught as such.
Once we have our Item ID, we can use that to create... a widget inside of a widget! That being our Item Slot widget, which is used to display an individual item. You can see the code for that blueprint below.
While I'm jumping around a bit, this code is important. The top half is fairly simple, we use that ID picked up earlier in Fetch Item to get data from our data table which defines the details of each one of our items, such as name or cost. That -1 ID comes into play here, as if the item isn't valid we set all of our text to be empty to let us know that item is invalid. If it is a valid ID however, we set our text correctly based on the Data Table.
The bottom half is where the spending happens. This is linked to the 'buy' button on each item, which is defined in the item slot itself. This checks the price of the item (which we know from the ID needed to create an item slot). If the player has enough money which we check via an inequality, we spend that money with a simple function that removes the cost from a variable stored on the player character, along with printing some fun text. Text is also printed if the player does not have enough money.
Moving back to the shop widget itself, we take all those item slots and place them in a grid panel on the widget. The index is divided by 3 in order to get 2 items on each row which makes it look a bit more neat and even. Finally we bind our events for purchasing an item. While we can handle the money situation from the item slot itself (as we have details about price), the overall shop widget managed the inventory via this event which is fired off when an item is bought in the item slot.
Status | Prototype |
Author | Thomas Sutton |
Leave a comment
Log in with itch.io to leave a comment.